I saw I had this blog post languishing on my desktop so I decided to post it.
There are hundreds of SEO tools out there that we can use, built by dozens of companies, but there is one tool that every SEO should use: Google Search Console (GSC). GSC is useful because it’s the only place where we can get search data directly from Google.
As many people know we can get this data directly from the web dashboard, but that comes with a lot of constraints, namely you can only export 1,000 rows at at time. Fortunately, there are a lot of ways around that these days, here are a few of them:
- Google Data Studio
- Keylime Toolbox
- Third party tools like Botify, Screaming Frog, and others export this data into their tools for you.
All of these are decent options, but none of them give you the flexibility of being able to go directly to the source, the GSC API. You’ll get more data and a say in how you store that data. On top of that, and perhaps the most valuable reason to use the API, is you’ll learn much more about the data within the tool.
For unfamiliar with the deeper aspects of Google Search Console, it can seem incredibly innacurate at times. There’s a lot of reasons for this such as data sampling, query dimensions, and how Google actually puts together the metrics in GSC. What’s great about the API is that you have to interact with this data at the most basic level so you’ll have a much greater understanding of the data coming out of GSC.
This article is meant to be a primer to the GSC API to show how simple it is to use it. When I was learning how to use GSC as a beginner programmer (I’m still a beginner now, but I was then too – credit @MitchHedberg) it was extremely confusing because all of the example scripts I could find on how to the API looked like this:
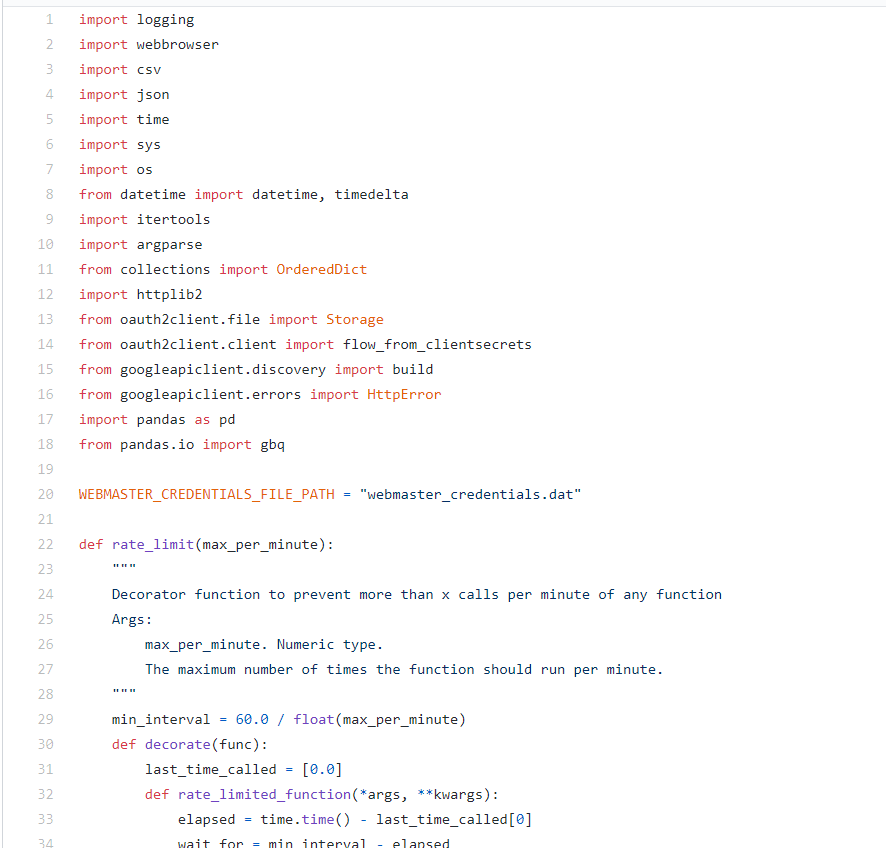
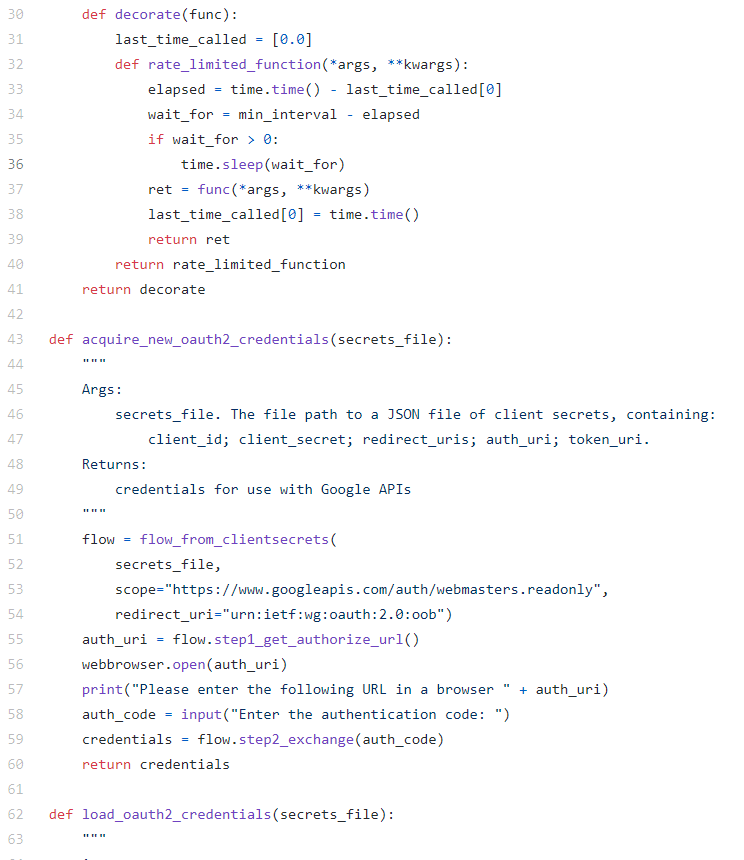
And that’s just a small piece of it, it goes on for another 120+ lines. That’s not to say this is a bad piece of code, to the contrary this example is really awesome once you understand what it’s doing. But it’s not great for beginners to understand how to use the API
GSC Query
I’m a firm believer that when learning how to use code, you need to start at the absolute basics. So with that in mind, I built the simplest possible GSC API query I could come up with so anyone who wants to learn should have an easy time figuring it out.
from googleapiclient import sample_tools
def get_gsc_data(property_uri, start_date, end_date):
service, flags = sample_tools.init([property_uri], 'webmasters', 'v3', __doc__, __file__, scope='https://www.googleapis.com/auth/webmasters.readonly')
request = {
'startDate': start_date,
'endDate': end_date,
'dimensions': ['query'],
'rowLimit': 5
}
response = service.searchanalytics().query(siteUrl=property_uri, body=request).execute()
for row in response['rows']:
print(row['keys'], row['clicks'], row['impressions'], row['ctr'], row['position'])
#VARIABLES:
gsc_property = "INSERT YOUR WEBSITE HERE"
gsc_start_date = "2020-01-01"
gsc_end_date = "2020-01-01"
get_gsc_data(gsc_property, gsc_start_date, gsc_end_date)
That’s as simple as it get’s right there. 16 lines of working code and 4 lines of variables to edit the script to your purposes. Here’s a link to the Github, if you’d prefer to clone a copy on your own Github account: https://github.com/baresluca/search-console-query-simple/blob/master/search-console-pull.py
This query first get’s the authentication information it needs to make a GSC API query and then it actually executes the query which is under the request variable. Finally we make an API call using the methods within the response variable and print them out line by line using a for loop.
What you really need to know is how to edit this script to fit your needs. The main pieces of code you’ll need to changes are the last 4 lines in this script which change the actual property and dates that you’re querying and the request variable which asks for the specific information to pull out of GSC.